The Singleton Design Pattern in Software Development
A singleton is a software design pattern that restricts the instantiation of a class to one object.
In other words, only one instance of the singleton class can ever be created. There are many reasons why you might want to use a singleton in your software development project.
In this article, we will introduce you to the singleton pattern and discuss when and how you should use it in your projects. We will also show you how to create a singleton class in Ruby.
Let's get started!
Introducing the Singleton Pattern
The singleton pattern is one of the most commonly used design patterns in software development.
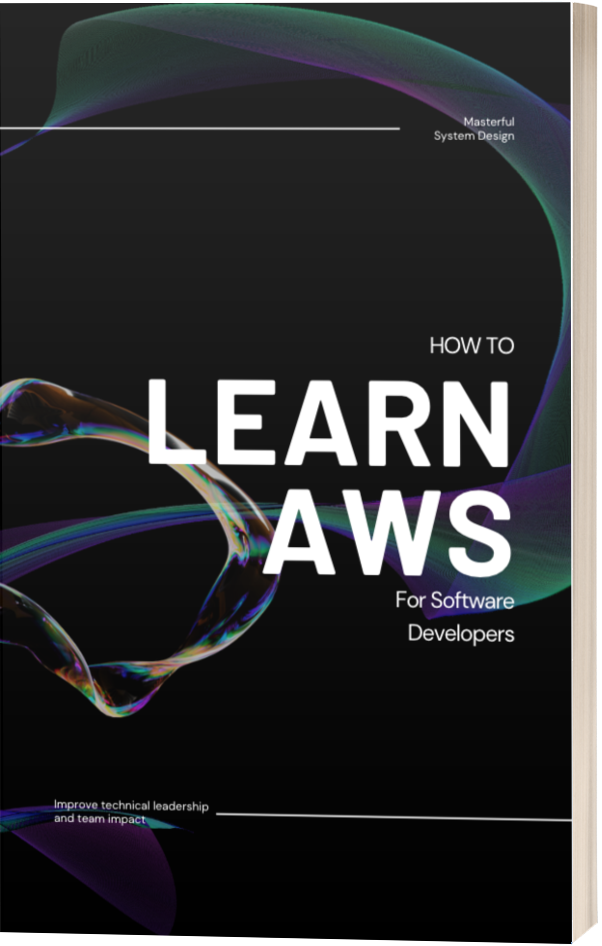
It restricts the instantiation of a class to only one object.
There are many reasons why you might want to use a singleton in your project. Some common reasons include:
- You want to control the number of instances of a given class that are created.
- You want to provide global access to an instance of a given class.
- You want to ensure that a specific instance of a class is used throughout your code.
When to Use a Singleton
When should you use a singleton? The singleton pattern should be used when you need to ensure that only one instance of a given class is ever created. Some common use cases for the singleton pattern include:
- Managing database connections
- Managing configuration options
- Logging messages to a central location
How Do You Create a Singleton?
We'll use Ruby for this example as creating a singleton in Ruby is relatively simple. To do so, you simply need to add the following code to your class definition:
class MyClass
def self.instance
@instance ||= new
end
private_class_method :new
end
By moving new
to a private method, we restrict the ability to create multiple instances of this class.
Attempting to instantiate a new instance of this class would raise an error.
MyClass.new
=> NoMethodError: private method `new' called for MyClass:Class
Instead of creating new instances, because this is a Singleton class we can call the instance
method we defined above to access the Singleton instance from anywhere in our codebase.
MyClass.instance
=> #<MyClass:0x00007fb45c8c6c80>
By memoizing the value our self.instance
class method returns, we essentially cache the first instance created by the new
method and just keep returning that same instance on subsequent calls.
MyClass.instance
=> #<MyClass:0x00007fb45c8c6c80>
MyClass.instance
=> #<MyClass:0x00007fb45c8c6c80>
In practice, the Singleton pattern is so common that Ruby provides a module you can import and use that does everything outlined above.
require 'singleton'
class MyClass
include Singleton
end
This code will ensure that only one instance of the MyClass class is ever created. You can then access this instance by calling the MyClass.instance method.
When Not to Use Singletons
There are a few things to keep in mind when using singletons in your software development projects.
First, you should be careful not to overuse singletons. If you find yourself using singletons in every class, then it's likely that you're not using them correctly.
Also, you should be aware of the implications of using global state in your application. When using singletons, you are effectively creating global variables. This can make debugging and testing your code more difficult.
What if most of your classes depended on the same Singleton class? Add a few new methods and attributes to it here, a few more for this other feature, and before you know it you have a 10K line class that breaks tests anytime you change anything.
That is a misfortune I wouldn't wish upon my greatest enemies.